In this article, we will be going through a way to call function declared in android code from javascript frameworks like angular or react or consider any javascript framework of your choice. Following are the various steps we are going to implement.
- Create an angular app and add it in android project through webView.
- Create functions to be called from javascript code in kotlin file.
Create an angular app
To create an angular app, you need node.js installed on your machine. Check this article to install node.js. Now, you need to install @angular/cli globally to access all ng commands. Open your terminal and run this command.
npm i -g @angular/cli
JavaScripton your desktop path, run this command to init angular app. Open webViewApp folder in your favourite code editor.
ng new "webViewApp"
JavaScriptOnce angular app is created, copy below code and paste it into app.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {}
title = 'angular-project';
callNative(){
//@ts-ignore
S1PWAClient.sendDataToNative("key", JSON.stringify({data: "lorem ipsum"}));
}
}
In app.component.html, replace all code with below code :
<button (click)="callNative()">CALL NATIVE FUNCTION</button>
Before running this angular app, we need to tell angular app to host it on current ip address. To find your ip address check this article.
Once you find your ip address, go to package.json file in your angular project. In the start script, change the default “ng serve” with ng serve –host “your ip address”. Now your script object should look like this:
"scripts": {
"ng": "ng",
"start": "ng serve --host 0.0.0.00/0", //your ip adress after host
"build": "ng build",
"watch": "ng build --watch --configuration development",
"test": "ng test"
},
JavaScriptYou can now start angular app with ng serve –o command and check on browser if app is up and running. If it is, copy full url of the app (for example: http://192.168.1.2:4200), we need it in next step.
Create an android app
Create a new empty activity project in your android studio. Choose kotlin as the language. Keep all the rest options as it is. click finish and you will be redirected to project window. In your “MainActivity.kt” kotlin file, paste the following code.
package com.example.myapplication
import android.content.Context
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.webkit.JavascriptInterface
import android.webkit.WebView
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
class MainActivity : AppCompatActivity() {
private val webViewUrl = "angular app url"//"http://182.153.1.2:4200/"
private lateinit var webPwa:WebView
private lateinit var editText:EditText
lateinit var string: String
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
webPwa= findViewById<WebView>(R.id.webPwa)
webPwa.settings.javaScriptEnabled = true
webPwa.addJavascriptInterface(JSClient(this),"S1PWAClient")
webPwa.loadUrl(webViewUrl)
}
class JSClient(private val context: Context): JsBridge {
@JavascriptInterface
override fun sendDataToNative(key: String, value: String) {
println(value);
Toast.makeText(context, value.toString(),Toast.LENGTH_SHORT).show()
}
}
}
KotlinJust change the value of webViewUrl to the copied url of your angular app. This way, our angular app is integrated as a webView in android app. Now, In activity_main.xml, paste following code :
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<WebView
android:id="@+id/webPwa"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Hello World!"
/>
</LinearLayout>
At this point, if you run android project you should be seeing something like this :
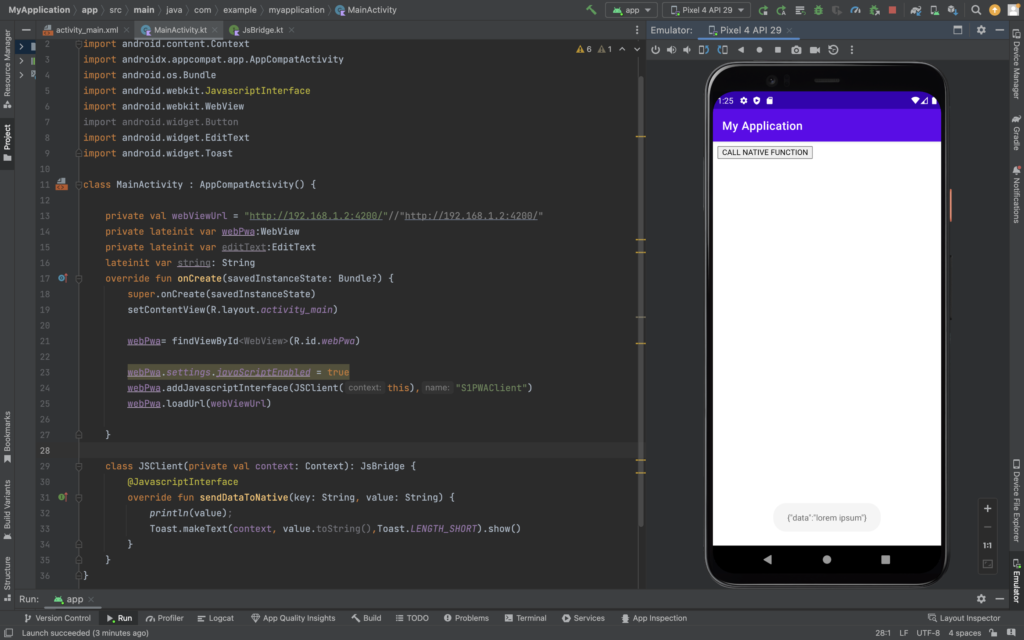
That’s it, whenever you click on “CALL NATIVE FUNCTION” button, callToNative function which resides in android studio will get called with whatever data you are passing from javascript. Instead of angular app, you can also use Vanilla javascript or React app.
Write more; that’s all I have to say. It seems as though you relied on the video to make your point. You know what you’re talking about, why waste your intelligence on just posting videos to your blog when you could be giving us something enlightening to read?